At the beginning of this year I started learning Vue 3, I had worked with version 2 before and the new version allows us to still use the same syntax, besides we can use the new Composition API, which is more powerful and customizable when creating reusable elements. In this post I will show how to create a new project with Vue 3 + Typescript and setting up ESLint and Prettier for working properly together in Visual Studio Code.
Vue CLI
The easiest and fastest way for creating a new project is using Vue CLI. It will allow us to set up the project using different predefined workflows. First of all we will install Vue CLI:
yarn global add @vue/cli
# OR
npm install -g @vue/cli
Once installed we can create a new project:
vue create hello-world
We will select the last option from the list:
Vue CLI v4.5.13 ? Please pick a preset: (Use arrow keys) Default ([Vue 2] babel, eslint) Default (Vue 3) ([Vue 3] babel, eslint) > Manually select features
And we can customize our project with the options present in the list. I chose the next ones, I didn’t select testing in order to focus on the topic:
Vue CLI v4.5.13 ? Please pick a preset: Manually select features ? Check the features needed for your project: (*) Choose Vue version (*) Babel (*) TypeScript ( ) Progressive Web App (PWA) Support >(*) Router ( ) Vuex ( ) CSS Pre-processors (*) Linter / Formatter ( ) Unit Testing ( ) E2E Testing
At the next step we will have to select more things, we choose version 3 and ESLint + Prettier at the end.
Vue CLI v4.5.13 ? Please pick a preset: Manually select features ? Check the features needed for your project: Choose Vue version, Babel, TS, Router, Linter ? Choose a version of Vue.js that you want to start the project with 3.x ? Use class-style component syntax? No ? Use Babel alongside TypeScript (required for modern mode, auto-detected polyfills, transpiling JSX)? Yes ? Use history mode for router? (Requires proper server setup for index fallback in production) Yes ? Pick a linter / formatter config: ESLint with error prevention only ESLint + Airbnb config ESLint + Standard config > ESLint + Prettier TSLint (deprecated)
After selection Vue CLI will start installing the dependencies. Once finished we can run npm run serve
so that our
new application will launch in development mode.
ESLint + Prettier
The Vue team has done a great job in order to avoid us headaches setting up ESLint and Prettier. If we open
.eslintrc.js
file we will see these plugins installed:
|
|
This way Prettier won’t conflict with ESLint rules. At lines 19-20 there are custom rules added by me, we can add what we want in order to customize it.
VSCode
Last but not least, we will configure VSCode in order to have everything working properly. The most important thing
is having installed the Vetur extension, which gives us a great development experience with Vue in VSCode. It will
give us auto formatting code in Single File Components (files with .vue
extension).
Once installed, we will go to Vetur settings and in Format -> Default Formatter
section we will select the formatters
for HTML, CSS, JS, Sass, Typescript… It is very important to select prettier-eslint for JS, I have the default
option in the rest including Typescript, with just Prettier instead of prettier-tslint which is deprecated.
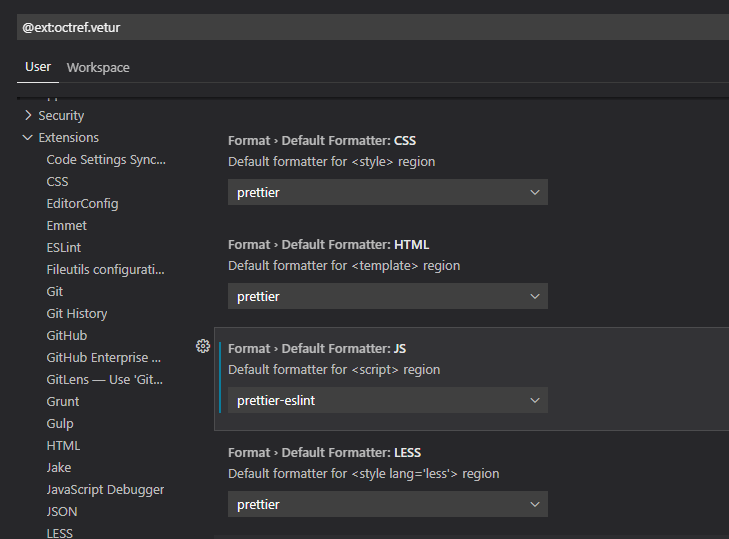
In VSCode settings we must add Vetur as default formatter for Vue:
"[vue]": {
"editor.defaultFormatter": "octref.vetur"
}
This way VSCode delegates in Vetur, and Vetur will format each different language that finds inside .vue
files with
a proper formatter. In my experience, there is only one thing wrong with auto format in .vue
files,
inserting the final new line at the end of the file. If you use this rule (which is the default), the only way I
could find is delegating this task in VSCode with this setting:
"files.insertFinalNewline": false
Sometimes using the last version of Vetur can be problematic, recently in a project Vetur started formatting HTML in a different way. After struggling for some hours I realized Vetur had been updated and after downgrading Vetur version everything started working as expected. I reported the bug and took the opportunity to comment on the problem with the final new line. Maybe I made a mistake, but I couldn’t find the problem after several hours.
After completing all the steps we are now ready to begin new projects with Vue 3 + Typescript + ESLint + Prettier in VSCode.